This demo shows how you can implement bottom sheets in flutter which works for both Android and iOS.
The StatefulWidget has a built-in function called “showModalBottomSheet’ which we will implement to show a bottom sheet.
Watch Video Tutorial
Modal Bottom Sheet
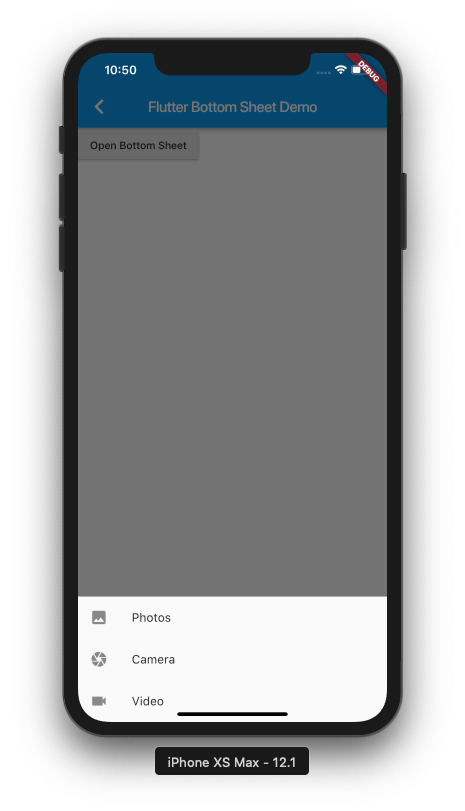
Modal Bottom Sheet in Flutter
The below function implements a bottom sheet which shows like a modal, that means it will be dismissed when you tap anywhere on the screen outside the sheet.
openBottomSheet(BuildContext context) { showModalBottomSheet( context: context, builder: (BuildContext context) { return Column( mainAxisSize: MainAxisSize.min, children: <Widget>[ ListTile( leading: Icon(Icons.photo), title: Text("Photos"), onTap: () {}, ), ListTile( leading: Icon(Icons.camera), title: Text("Camera"), onTap: () {}, ), ListTile( leading: Icon(Icons.videocam), title: Text("Video"), onTap: () {}, ), ], ); }); }
This will take the BuildContext parameter which you can pass from the build function.
Persistent Bottom Sheet
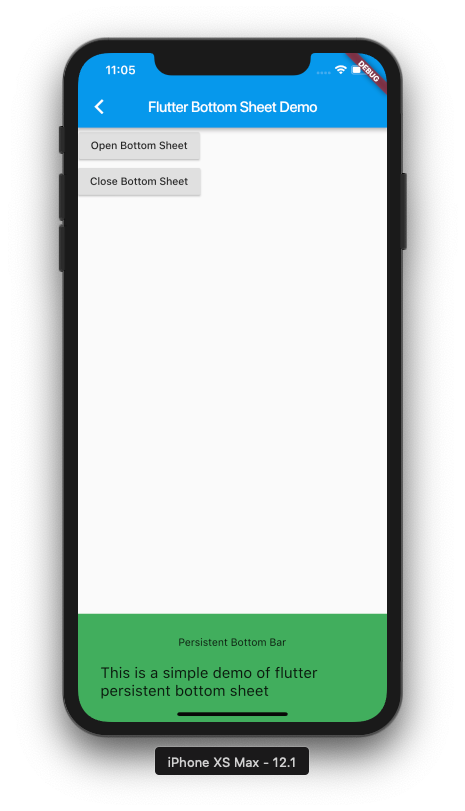
Persistent Bottom Sheet in Flutter
We have to create a GlobalState<ScaffoldState> and a PersistentBottomSheetController instance to create a persistent Bottom sheet across the screens.
Declare the variables
final scaffoldKey = GlobalKey<ScaffoldState>(); PersistentBottomSheetController controller;
Let’s write the function that shows a Persistent Bottom Sheet.
openPersistentBottomController(BuildContext context) { controller = scaffoldKey.currentState.showBottomSheet((BuildContext context) { return Container( padding: EdgeInsets.all(30.0), color: Colors.green, child: Column( mainAxisSize: MainAxisSize.min, children: <Widget>[ Text("Persistent Bottom Bar"), SizedBox( height: 20, ), Text( "This is a simple demo of flutter persistent bottom sheet", style: TextStyle(fontSize: 20), ), ], ), ); }); }
Don’t forget to add the key to the Scaffold that is calling this function.
@override Widget build(BuildContext context) { return Scaffold( key: scaffoldKey, ...
Make sure you assign the controller…
controller = scaffoldKey.currentState.showBottomSheet((BuildContext context) { ....
That’s it. It’s that simple in Flutter.